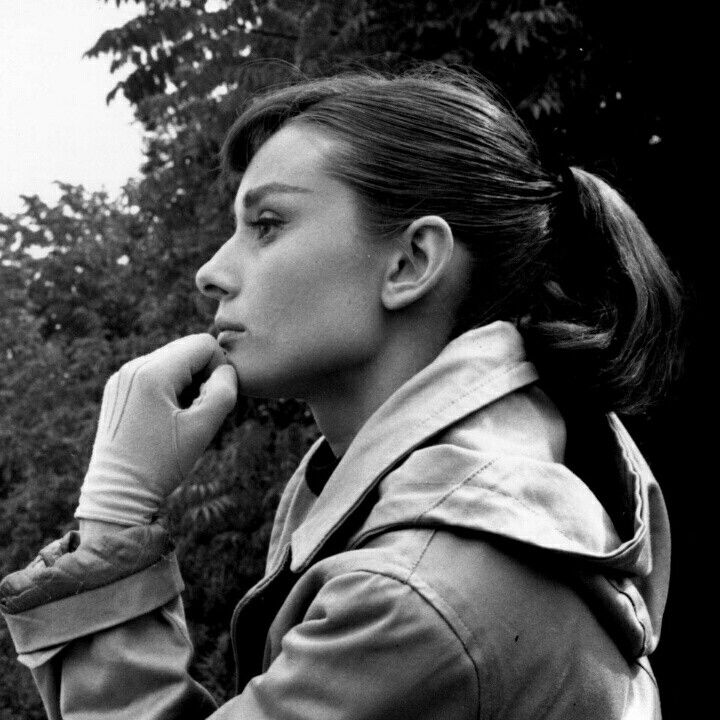
[toc]
GCD(1)任务的顺序
前言
GCD这个东西老生常谈了,我们在开发中还是比较常用,几乎我们每个项目中都会用到,但是频率却又不是特别高(先别反驳)。
因为可能只是个别对操作有特殊要求的地方使用,所以导致很多人对GCD熟悉但是不熟练,你可能会说我们的网络请求,图片加载等都用到了GCD实现多线程啊,是的不错,不过这些都是框架比如afn,sd等给我们封装好了的,真正我们主动自己去封装GCD进行操作的地方并不是很多,包括我自己之前对其中的部分也不是特别明白,后面我会模拟一下我们常用的操作,来让我们正确通过GCD使用多线程。
代码
国际惯例先看几个示例:
示例1
下面的代码打印顺序是什么样的
1 | dispatch_queue_t queue = dispatch_queue_create("lazy", DISPATCH_QUEUE_CONCURRENT); |
我们先不着急给答案,先给答案可能会先入为主,影响理解,先来分析,看看答案可能是什么
分析:
queue
是一个并发队列,任务12356都在这个队列,并发队列有多个任务出口,任务4在主队列,主队列是串行队列,只有一个任务出口queue
中任务3是个同步任务,特点是不会开辟线程,且会立即执行任务并阻塞线程直到任务结束才执行下一个任务,1256是异步任务,可以开辟线程,不会立即执行会等待CPU调度,并且不会阻塞线程- 通过前两步分析可知,
queue
中3不执行完,后续任务不会进入队列,那么56肯定在3之后入队列,而12入队列在3之前但是是异步任务,执行顺序不确定,所以12可能在3之前也可能在3之后,也可能3在12之间,那么其实1256整体之间的顺序就也不确定,4虽然不在queue
中,但是3阻塞了主线程,所以4也在3之后 - 3结束后立马执行了4,56因为是异步任务顺序也不确定,但肯定在4之后
经过上面的分析可以得出几个可能的答案,分别是:
- 123456
- 324156
- 312465
…当然还有其他符合的答案,具体根据代码运行时CPU的状态决定,但都会符合上边4条分析的结论,下面我贴一下我这边打印出的几个结果
1 | 3--<_NSMainThread: 0x600002ed0140>{number = 1, name = main} |
你也可以亲自动手试一下
示例2
下面的代码打印顺序是什么样的
1 | dispatch_queue_t queue = dispatch_queue_create("lazy", DISPATCH_QUEUE_CONCURRENT); |
同样的我们先来分析,看看答案可能是什么
queue
是一个并发队列,234是异步任务在这个队列中,15在主队列(上边重复过的内容省略)- 1肯定先打印,其他任务都在它之后,之后24加入队列,因为这是一个异步任务所以会先入队列等待执行,之后打印5
- 而当异步任务块被执行时(注意这个执行时),打印了2之后,又有一个异步任务3,此时将任务3加入队列等待执行,然后继续执行4,所以34肯定在2之后
- 那么24这个异步任务块结束后会从队列中取出3执行,为什么会等24之后执行3呢?因为3是在24执行时加入队列的任务,它入队列时候已经在执行打印24了,而3还处于等待执行状态
- 最后打印出来就是15243
1 | 1--<_NSMainThread: 0x600003224140>{number = 1, name = main} |
那么下面再稍微变一点点,再看打印的是什么
1 | dispatch_queue_t queue = dispatch_queue_create("lazy", DISPATCH_QUEUE_CONCURRENT); |
我们来分析下
queue
是一个并发队列,24是异步任务在queue
中,3是同步任务在queue
中,15在主队列(上边重复过的内容省略)- 1肯定先打印,其他任务都在它之后,之后24加入队列,因为这是一个异步任务所以会先入队列等待执行,之后打印5
- 当异步任务块被执行打印了2之后,此时有一个同步任务3加入队列,那同步任务的特点是不开辟线程并阻塞线程立即执行,所以3会打印,然后继续执行4
- 最后打印出的结果就是15234
1 | 1--<_NSMainThread: 0x600003a2c7c0>{number = 1, name = main} |
示例3
下面的代码打印顺序是什么样的
1 | dispatch_queue_t queue = dispatch_queue_create("lazy", DISPATCH_QUEUE_SERIAL); |
可以看出,这个和上边的示例也很相似,我们先来分析下
queue
在这里是串行队列,24是异步任务在queue
中,3是同步任务在queue
中,15在主队列(上边重复过的内容省略)- 1肯定先打印,其他任务都在它之后,之后24加入队列,因为这是一个异步任务所以会先入队列等待执行,之后打印5
- 当异步任务块被执行时打印了2之后,此时有一个同步任务3加入队列,那同步任务的特点是不开辟线程并阻塞线程立即执行,可是
queue
是个串行队列只有一个出口也就是同一时间只能有一个任务在出队列,那么在24出了一半的时候,3要求立马出可是3在队列中处于24之后,就造成了拥挤等待,24执行完才能执行3,3要求立马执行,执行完才能执行4,互相等待就会crash - 所以打印应该是152然后crash
这种相互等待的情况就叫死锁,所以我们在使用串行队列,并且有同步任务的时候,一定要注意,在同步任务执行前,一定确保之前的任务都执行完了
示例4
那么看了上一个死锁导致crash的例子后我们再来看一个
1 | dispatch_queue_t queue = dispatch_queue_create("lazy", DISPATCH_QUEUE_SERIAL); |
国际惯例先来分析
queue
是个串行队列只有一个出口也就是同一时间只能有一个任务在出队列,后边的任务不能插队,23都在queue
这个队列中,14在主队列(上边重复过的内容省略)- 14都在主队列,1肯定先打印,234都在它后边,那4呢?4之前有个同步任务3,通过上边几个例子我们知道同步任务的特点,所以4肯定要等3执行完,那么4就在3之后,
- 再来看2,它虽然是一个异步任务,但是串行队列只有一个出口也就是同一时间只能有一个任务在出队列,先加入队列的任务即使不执行也会挡住出口,
queue
中3排在2之后,3要等待2执行完才能开始执行 - 那么这个例子和上边死锁的有什么区别?这例子中3虽然是同步任务但并不是在2执行时加入队列的,所以2执行时不需要考虑3的情况,也就不会造成相互等待,也就不会死锁
- 那么分析后最终应该是1234
1 | 1--<_NSMainThread: 0x600002538440>{number = 1, name = main} |
如果对于第3条分析里2这个异步任务的顺序有疑问,可以吧代码中2上边的注释sleep(1)
打开,看是不是即使2睡了一秒后,3还是会在他之后
示例5
再来看最后一个,下面的代码循环外会打印什么?
1 | __block NSInteger num = 0; |
同样的我们先来分析
- 一个while循环,出循环的条件是num >= 10,并且是在主队列主线程执行
- 循环里边会将一个异步任务加入全局队列,任务是将循环外的变量num加1我们知道全局队列是一个并发队列,这里你也可以像上边的例子一样自己创建一个并发队列
- 并发队列有多个出口,允许多个任务同时执行,异步任务不会阻塞线程并且会先加入队列等待调度执行
- num只有在while循环里边才会进行+1,所以最少要加10次才会变成10,也就是最少会有10个异步任务被加入队列
- 但是通过第3步我们知道任务进去后不一定立马被执行,可能一个num++任务加入队列后还没来记得执行,while已经开始了下一次循环又将一个任务加入队列(取决于你的CPU状态),所以num可能被额外的++,而循环可能在某次++后跳出,但是队列中可能还有++任务未被调度
- 那么最低次数 10 + 额外次数 一定大于等于10,结果应该就是大于等于10
来看一下打印
1 | 1--<NSThread: 0x6000028a58c0>{number = 4, name = (null)} |
可以看出循环外的log打印的是12,多余的打印就是额外加入队列的任务
后记
通过上边几个例子,我想你对于GCD的使用应该更加的熟悉了,但是这里只是列举了几种使用相对简单的场景,我们实际中可能需求更复杂,比如多个网络请求结束后执行某些操作,或者多个请求之间有依赖,或者对于某些数据要实现多读单写等,这里是抛砖引玉,先对基本使用有更深刻的理解,然后后续会结合更复杂的场景来介绍
- 本文标题:GCD(1)--任务的顺序
- 本文作者:Lazyloading
- 创建时间:2022-05-26 17:50:54
- 本文链接:https://lazy.wiki/posts/a3a7c4e/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!